As the next step in my recent adventures into NoSQL country I decided to take a look at db4o. Shortly put I hope it will give me more than the document based databases, like Mongo that I have also been taking out for a spin.
My interest in NoSQL has been kindled further than it already was because of blog posts from Rob Connery, who looked into practical approaches and posts on CodeBetter provokingly entitled Using an ORM is like kissing your sister. Basically what they are all saying is that it is very limiting that we always start out with a relational database without even considering this very very important architectural decision.
Object vs Document
Similar to the document based database, the big win is that there is no schema, and no need to map between objects and a relational model. Actually with document based databases you do map to key/value pairs, so it’s not the whole truth, but still simpler than mapping to a relational model.
Mapping can be expensive in two ways – because it takes time to write the code, and it does warrant a certain overhead. On top of that db4o as an object oriented database has a concept of relations between objects. This means that rich querying capabilities are build in and that it performs really well.
When comparing to MongoDB the big thing missing is that it basically works on a flat file out of the box. So you need something to handle concurrency and stuff like that. Rob Connery posted a nice sample of how this could be done, and its really quite straight forward, because db4o does provide all you need to do it.
The basic stuff
In the interest of simplicity I’ll just sample how to work with db4o directly. Also this will illustrate just how straight forward it really is. The first thing that brought a smile to my face was how little I had to do to save and query a bunch of objects.
using (IObjectContainer context = Db4oEmbedded.OpenFile(Server.MapPath("myDatabase.yap")))
{
var empolyeeToSave = new Employee() { Name = "John", Card = new TaxCard() { Number = "12345678" } };
context.Store(empolyeeToSave);
IList<Employee> retrievedEmployees =
context.Query<Employee>(employee => employee.Card.Number == "12345678");
}
As you can see it’s very straightforward stuff. Besides this type fo querying which takes a predicate, there is also a QueryByExample, which works as one would expect. Another nice thing is that all calls to the Store method make up a transaction, so there is a Rollback method available. When the context is disposed the convention is that Commit is called for you, so when you don’t need rollbacks you don’t have to think about it.
Indexing is also possible with db4o, and it is also very simple to do, so if for instance I would like to apply an index for the name property on my Employee I could do this.
Db4oFactory.Configure().ObjectClass(typeof(Employee)).ObjectField("Name").Indexed(true);
Moving along
Some of the first things that I have heard people think about when I say object/document based database is how to handle versioning and querying. With querying in this case I mean how to enable a scenario similar to opening SQL Manager and writing some queries to take a look at the actual data.
The versioning part may take a little work, but there are methods to help with e.g. renaming directly baked in - and besides, it takes work no matter what kind of database your using. On top of that my thought is that both of these issues can be addressed with a dynamic language. My plan is to take a look at this at some point, and that it will fit nicely because my copy of IronRuby Unleashed should be on it’s way :)
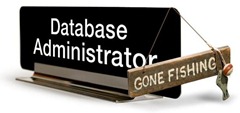