Providing a customized high quality user experience is becomming increasingly important on the web. It is no longer enough to provide information and functionality, but it also has to look and feel nice in a way that contributes in building the company brand. To do this we needed to shift some of the focus toward what is running on the client.
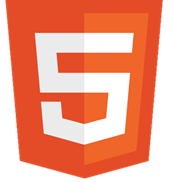
Fattening up the client
At first the search for fatter and richer clients lead us to focus on Flash or Silverlight. While providing a rich design experience this also comes with its own set of limitations. Limitations such as requiring users to install a browser plugin that in turn removes the browser experience with linking and the options to copy text and images. Since then we have come full circle so we once again use *drumroll* JavaScript to power applications. With the emergence of HTML5 and CSS3 that will be running across computers, tablets and phones it looks like JavaScript and friends will continue to play a key role in developing tomorrows applications.
Curing the JavaScript headacke
A few years ago I - like most developers at the time - did not like JavaScript. We saw it as a necessary evil to allow validation, and something we needed to fight with once in a while to show/hide elements on webpages. JavaScript suffered from a range of illnesses such as browser incompatibility, performance issues, lack of widely adopted development practices and a rap for being brittle.
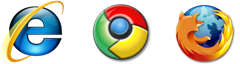
Along came jQuery - a JavaScript library that did a good job at shielding the developer from browser incompatibility while providing a simple API for doing DOM manipulation and providing structure for plugins to be built. The library has since become so popular that some developers talk about "writing jQuery" rather than JavaScript.
Thanks to the need for better user experiences, jQuery and in no small part the technological advances in the browser reguarding performance, we developers spend more and more time writing JavaScript. While jQuery has helped a great deal there are still areas where working with JavaScript seems unstructured and primitive in reguards to expressiveness and robustness. So lets look at some options we have to accomodate those needs.
Underscore - the functional tie
Underscore is a JavaScript library that prides itself in being "the tie to go along with jQuery's tux". Basically it provides a utility-belt for doing functional-style programming in JavaScript. This is important because regular JavaScript constructs tend to be so low-level that "what" you are doing is drowning in "how" you are doing it. With underscore you get quite of bit more expressiveness, making code easier to read and maintain. Besides the functional core underscore also provides a way through some shortcommings of JavaScript - like binding functions to objects - and it has templating capabilities.
This sample shows off some of the functional capabilities, as well as how to use templates.
var sumOfEvens = _(_.range(1, 50))
.chain().map(function(number) {return number * 2;})
.reduce(function(sum, number) {return sum + number;})
.value();
var template = _.template("The sum of even numbers between 1 and 100 is <%= sum %>");
console.log(template({sum : sumOfEvens}));
"Grow a spine"
Spine and Backbone are two very similar frameworks that provide structure to applications written in JavaScript through an MVC pattern. In more and more scenarios each page can be seen as a small application by itself, so structure is becoming as important as it is on the server. Spine is the smallest of the two, so I will focus on that - but mostly you can assume that Backbone works pretty much the same way. Fundamentally they allow you to work with classes so you get a way to do inheritance. Building on that you can create models for persisting data and controllers (which are called views in backbone) that give you a structure for defining events and functions. To support this the libraries also have functions for working with events and a way to handle routing with hashtags.
This sample shows how to work with a model that is persisted to local storage, how to handle events and how to respond to routing.
var Contact = Spine.Model.setup("Contact", ["id", "first_name", "last_name"]);
Contact.extend(Spine.Model.Local); //Saves to local storage
var eric = Contact.init({id: 1, first_name: "Eric", last_name: "Cantona"});
eric.bind("save", function(){ console.log("Saved!"); });
eric.save();
var ryan = Contact.init({id: 2, first_name: "Ryan", last_name: "Giggs"});
ryan.save();
var App = Spine.Controller.create({
init: function(){
this.routes({
"/users/:id": function(id){
var c = Contact.find(parseInt(id));
console.log("Name: " + c.first_name + " " + c.last_name);
},
"/users": function(any){
Contact.each(function(c){
console.log("Name: " + c.first_name + " " + c.last_name)
});
}
});
},
events: {"click input": "click"},
click: function(event){
var c = Contact.find(1);
console.log("Name: " + c.first_name + " " + c.last_name);
}
}).init({el: $("#Players")});
Spine.Route.setup();
I have previously written a bit about KnockoutJS, which can be seen as an alternative to using Spine or Backbone. Rather than provding a MVC-like structure Knockout allows you to work with a MVVM model with two-way databinding. I will not try and argue that the Spine/Backbone or KnockoutJS approach is "better", but leave you with the typical developer cop out "it depends". The design decision that you face really is, what brings greater value in your case, modularity, eventhandling and routing or two-way databinding, templating and dependency tracking?
"Testing testing 1-2-3 - is this thing on?"
The last piece of the puzzle is to introduce testing which should help us write and maintain more robust applications. QUnit is a simple unit testing framework that is popular amongst the people who work with jQuery and plugins for jQuery. QUnit lets you group tests into modules, so you get a nice overview when you run the testsuite.
add = function(a, b) {return a + b};
module("Math module");
test("addition", function() {
expect(2);
equal( add(2, 2), 5, "failing test" );
equal( add(2, 2), 4, "passing test" );
});
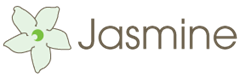
For those who prefer a BDD style framework Jasmine is a popular choice. Besides having a different style Jasmine also has functionality to work with spies, for some more advanced testing scenarios. Both frameworks provice a clean and easy to read syntax, so choosing between the two comes down to taste or if there is some small feature in either that you like.
function Calculator() {}
Calculator.prototype.add = function(a, b) {
this.log(a + " + " + b + " = " + (a + b));
return a+b;
};
Calculator.prototype.log = function(text) {
console.log(text);
};
describe("Calculator", function() {
var sut;
beforeEach(function() {
sut = new Calculator();
});
it("should be able to add", function() {
var result = sut.add(2, 2);
expect(result).toEqual(4);
});
it("should log what is added", function() {
spyOn(sut, 'log');
var result = sut.add(2, 2);
expect(sut.log).toHaveBeenCalledWith("2 + 2 = 4");
});
});