by DotNetNerd
14. December 2022 12:32
Recently I needed to update Azure Management API based on the swagger specifications when our services were deployed. It seems like a pretty standard thing that there would be a task for, but it turned out to require a bit of Powershell - it i still fairly simple though.
A general function to acccomplish it could look like this bit of code.
[CmdletBinding()]
Param(
[string] [Parameter(Mandatory=$true)] $ResourceGroupName,
[string] [Parameter(Mandatory=$true)] $ServiceName,
[string] [Parameter(Mandatory=$true)] $ApiName,
[string] [Parameter(Mandatory=$true)] $SpecificationFilePath
)
$apiMgmtContext = New-AzApiManagementContext -ResourceGroupName $ResourceGroupName -ServiceName $ServiceName
$api = Get-AzApiManagementApi -Context $apiMgmtContext -ApiId $ApiName
if ($null -eq $api) {
Write-Error "Failed to get API with name $ApiName"
exit(1)
}
$apiVersionSetId = $api.ApiVersionSetId.Substring($api.ApiVersionSetId.LastIndexOf("/")+1)
$apiVersionSet = Get-AzApiManagementApiVersionSet -Context $apiMgmtContext -ApiVersionSetId $apiVersionSetId
Import-AzApiManagementApi -Context $apiMgmtContext `
-SpecificationUrl $SpecificationFilePath `
-SpecificationFormat 'OpenApi' `
-Path $api.Path `
-ApiId $api.ApiId `
-ServiceUrl $api.ServiceUrl`
-ApiVersionSetId $apiVersionSet.Id
This can of course be used locally, but to run it from a release pipeline, the cleanest way I have found, is to add it to a separate repository, and include it as an artifact. From there we just need a Azure Powershell build step, configured as shown by the YAML below.
variables:
swaggerUrl: 'https://my_appservice.azurewebsites.net/swagger/1.0.0/swagger.json'
steps:
- task: AzurePowerShell@5
displayName: 'Azure PowerShell script: Update API Management'
inputs:
azureSubscription: 'xxx'
ScriptPath: '$(System.DefaultWorkingDirectory)/_MyCompany.BuildScripts/UpdateApiManagement.ps1'
ScriptArguments: '-ResourceGroupName "resource_group_name" -ServiceName "management_api_service_name" -ApiName "unique_api_id" -SpecificationFilePath $(swaggerUrl)'
azurePowerShellVersion: LatestVersion
And that is all that is required, to automate it and make it update along with the build. Nice and easy.
by dotnetnerd
9. November 2018 11:25
Cloud native is one of those words that make some people shake their heads and call BS. In some contexts I am one of those people. It does however also have its place, because building solutions that are cloud centric does come with a number of benefits and enables solutions that were very hardif not impossible pre-cloud.
Sure, you can script the setup of a server from scratch, but it requires quite a bit of work, it takes time to execute and you still end up with an environment that requires updates and patching as soon as the script is a week old. In a cloud setup good practices, in the form of DevOps mainly using the CLI makes this very obtainable. Actually the current environment I am working with combines an ARM template and a few lines of script so we can spin up an entire environment in about 15 minutes. The only manual step is setting up the domain and SSL cert, but even that could be scripted if I wanted to.
More...
by dotnetnerd
5. October 2017 12:05
I recently started a new project, based on my own medicine of not using a framework, but building a simple architecture, that can grow and change freely based on needs as they arise. It is coming along very nicely, and has allowed us to have a pretty clean architecture.
For this project we chose to base the solution on TypeScript, and use handlebars for views along with a few other small libraries. As a clientside build tool we wanted to use webpack, because it is fast, has a lot of plugins that we can use, and is well suited for working with css modules. More...
by DotNetNerd
29. March 2016 12:59
A core value that Azure brings to modern projects, is to enable developers to take control of the deployment process, and make it fast and painless. Sure scalability is nice, when and if you need it, but the speed and flexibility in setting up an entire environment for your application is always valuable - so for me this is a more important feature of Azure. Gone are the days of waiting at best days, most likely weeks and maybe even months for the IT department to create a new development or test environment.
More...
by DotNetNerd
8. November 2015 14:12
With ASP.NET 5 which is currently in Beta 8, Microsoft has launched a new web server named Kestrel, which is of course Open Source. There are a number of reasons they are building Kestrel, but most importantly to provide a cross-platform web server which does not rely on System.Web and a full version of the CLR in order to bootstrap the new execution environment (DNX) and CoreCLR - which was not possible with Helios.
More...
by DotNetNerd
25. June 2015 07:40
Pleanty of people have said that concurrency and functional programming are becomming essential to developing modern systems. Some have even gone so far as to say that if you don't adapt you will become a maintenance programmer in a few years. With the increasing need for concurrency to provide speed and handeling of huge amounts of data, I beleive it to be true. I do however beleive there will still be pleanty of smaller applications, so I am not predicting doomesday for anyone quite yet.
More...
by DotNetNerd
4. May 2015 05:22
At Build there was a number of huge announcements. It is amazing to see that Azure is keeping up the pace, and as a developer I am excited about the promise of services that will enable me to focus on implementing solutions rather than fiddeling with servers and infrastructure.
More...
by DotNetNerd
1. May 2015 10:13
Besides App Services, Azure offers an alternative that is slightly to the left of the far right on the big IaaS to PaaS scale called Azure Service Fabric. Conceptually Service Fabric is based on containers, allowing you to fit many services into a single VM and it gives you more fine grained control than with App Services by providing a manifest describing how it should operate.
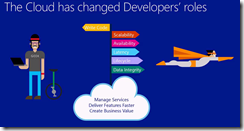
More...
by DotNetNerd
28. November 2014 13:43
Lately I have been speaking quite a bit about building Single Page Applications. Most recently I have been arguing that we skipped the discussion of weather or not we need a framework at all to build a Single Page Applications? Everyone quickly got into the battle of the frameworks, and argued about the good and bad parts of Angular, Ember, Durandal and a bunch of other contenders to the throne. These days I would argue that Angular won, which is supported by google batteling the frameworks, looking at training courses offered and joblistings. As a .NET developer this is furtner obvious now that AngularJS is part of the ASP.NET package, and samples are showing up that use it for Apache Cordova applications, which are also being embraced by Microsoft.
However, the more I have been working with these frameworks, the more I have started to question their actual value. At least to a point where I think it is something we need to think more about, when choosing which way to go. Sure frameworks give you a nice package that tackles application structure, routing, templating, two-way binding and dataaccess - but at what price? Using a framework, always means letting someone else decide a lot of things on your behalf, and it means taking a dependency on a large codebase. So in this blogpost, I will try and make the case for growing your own architecture.
You might think "so what, we do that all the time, we shoulden't be reinventing the wheel and what does it matter to depend on a framework". If they provide enough value I would surely agree, but it should always be measured against the risks of vendor lockin, issues with breaking changes, performance implications and restrictions on growing your application going forward. With a fast evolving web, these issues are very real. A framework might still be a good choice, but we need to at least think about the pros and cons.
Two-way binding is a huge sinner when it comes to performance and modelling restrictions, if the model chosen is not right for what you are building. Breaking changes are hurting developers daily, and with both Angular and Durandal looking to be complete rewrites for their next versions - so if you use them, you will be stuck with the current version, or you will be doing a rewrite of your own fairly soon.
More...
by DotNetNerd
26. September 2014 17:46
As I wrote before the conference I was glad to see some good speakers on the Microsoft track. So of course I prioritized a couple of these sessions, and went to Mads Torgersens C# talk and to Mads Kristensen talking about tooling in ASP.NET. Well technically Thorgersens talk was on the architecture track, but you get the point.
The new world after Roslyn
The C# talk went a bit more into writing analyzers and codefixes than what I had seen before, so that was quite interesting and one of the very concretely usefull takeaways. The idea is that writing an analyzer is just like working with any object model. No more magic, so writing extentions is becomming something any developer can do and no longer reserved for the minority with too much time on their hands. Torgersen also demoed some of the new language features that I blogged about earlier, but on top of that let slide that primary constructors probably won't make it into the language for the final release in the comming version. The reason is that they want to do more and look at providing recordtypes as well as pattern matching - and want to make sure that they get it right. These are two great features that I miss from F# on a daily basis, so I was glad to hear this. I also aired the idea of make shorthands for stuff like factory methods, which could also reduce some of the boilerplate code that I see a lot.
More...